Hello everyone, we have already set up the lab environment. In this article, we will discuss about the static analysis of the android APK.
What is an APK?
Before diving into the security stuff of the application, we need to understand the APK. Android Package Kit (APK) is the most common package file format used to distribute and install apps on Android devices. The APK contains everything that is necessary for the application to run. Some of the common contents in APK are:
- AndroidManifest.xml file describes the name, version, access rights, activities, and other useful information about the application.
- assets folder contains the application’s assets and resource files.
- META-INF folder contains the application’s certificates, signature, and a list of resources.
- resources.arsc file contains the pre-compiled resources used by the app.
- res folder contains the resources that are not compiled in resources.asrc.
- lib folder contains the platform dependent compiled code and native libraries for specific architectures i.e. x86, x64.
- classes.dex files are the compiled Java classes in DEX file format.
Whenever you install an application from the PlayStore, the device handles the installation through the APK file. However, for manual installation, you can download the APK first and then install it. But sometimes, the android device blocks the manual installation of APKs. For this, you need to Allow app install from unknown sources
from within the device’s settings.
APK Analyses
Mainly there are two types of APK analyses, Static and Dynamic. In this article, we will be focused on the static part of the analysis.
APK Dynamic Analysis
As the name suggests, the dynamic analysis deals with the analysis of the application while it is running. There are many factors to consider while performing the dynamic analysis. We will discuss dynamic analysis in detail in the coming articles.
APK Static Analysis
Before performing the dynamic analysis of the application, it is really important to do a static analysis. Static Analysis involves but is not limited to:
- Finding hard-coded credentials
- Checking for the permissions
- Application transport security
- Application activities
- API keys
- Code inspection and analysis
Also, for performing the static analysis, we don’t need to install the application on the device. However, sometimes the application creates some database files and other files immediately after installation. We will discuss this in the dynamic analysis part.
Manual APK Static Analysis
For the manual static analysis of the APK, we will use JADX GUI tool. Before that we need to get APK for an application. If you don’t already have the APK, you can download the one from APK Combo
. In APK Combo, you can put the PlayStore link and it will generate the APK download link. For the static analysis, we will be using Injured Android APK
. You can download the APK directly from this link. Alternatively, you can check the Github Repo as well.
Static Analysis using JADX
Now that we have the APK, open JADX GUI and you will see the following window screen:
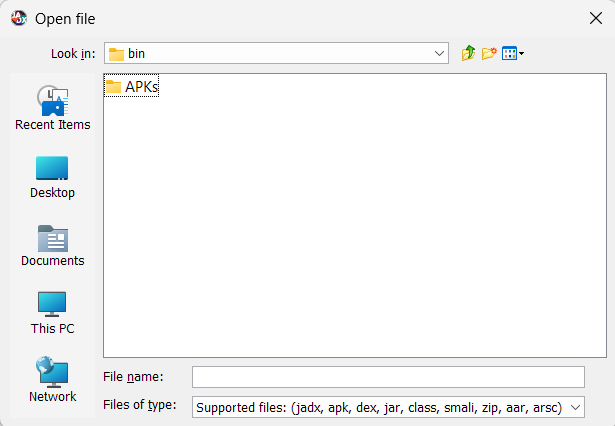
Choose the APK from the destination folder and double click APK to load into JADX.
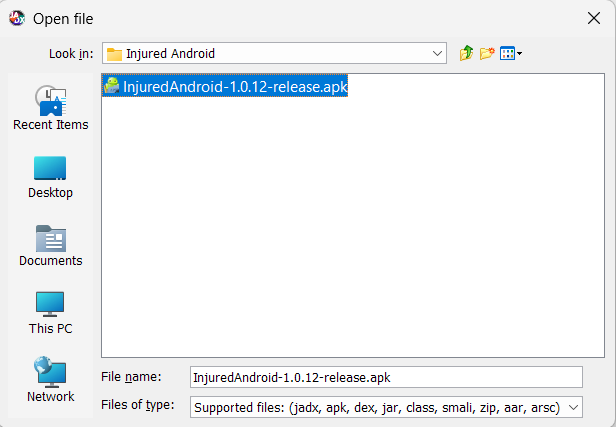
However, you can simply drag and drop the APK directly into JADX main window as well.
Eventually, JADX will decompile the APK and after some time, you will see the folders in left pane as below:

Inspecting AndroidManifest.xml
Very first file that we will examine is the AndroidManifest.xml file in Resources
folder.

Double click on the file and you will see its contents in the right pane as below:

This file contains the essential information about the application. It gives us the understanding of the application’s structure and functionality.
Package Name
It is important to find the application’s package name as the same name folder in Source Code
will contain the full or partial code for the application. You can find the package name in the manifest
tag with attribute package
as below:

Permissions
Upon installation, the app asks for the permissions. Many people give all those permissions to the application even without reading them. This sometimes also leads to their phones getting hacked. It is really important to check the permissions that the application is asking and then observing the permissions. For example, if a Flash Light application requires the gallery or camera permission(s), it clearly is something suspicious. You can see the permissions from android:uses-permission
tags as below:

You can find the list of the android permissions with their description here.
Network Security Config
This file contains the network configuration for the application. You can see the file path in the application
tag with attribute android:networkSecurityConfig
as below

You can find the file in res → xml → network_security_config.xml

The cleartextTrafficPermitted
attribute being true tells that the application allows the traffic over insecure protocol HTTP. This must be avoided in production environments but for testing purposes, it can be used in non-production environments.
Also, the applications with cleartextTrafficPermitted=true
are rejected by PlayStore as well for user privacy protection.
Backup
Not in this application, but sometimes the application
tag contains the attribute android:allowBackup
. This defines whether application data can be backed up and restored by a user who has enabled USB debugging. If this attribute is true, then attackers can take backup of the application data even with non-rooted phone.
Debuggable
Sometimes, the application
tag has the attribute android:debuggable
that defines whether the application can be debugged or not. If this attribute is true, the attacker can access the application data by assuming the role of the application. This may lead to even Remote Code Execution under that application’s permissions. However, for the non-debuggable applications, attacker will need to root the phone first before extracting any data. Therefore, it is also important for developers to properly implement device root checks in the application.
strings.xml
This file usually contains all the strings that the application will use. The primary purpose of this file is the localization. However, many developers store the sensitive strings in this file. Opening the res → resources.asrc → values → strings.xml
file, you will see various hard-coded strings:

Next we need to analyze this file’s contents to find any sensitive information. We can find the firebase
URL, that attacker can check for proper permissions, as below:

It is also common to store API keys in this file as we find google_api_key
, that attacker can check for the permissions via KEY HACKS for various APIs.

Developers sometimes also store the backend URLs, bucket URLs, and other sensitive strings, as below:

Code Inspection
It is important to inspect the code as well. You can find the source code in the Source Code
folder with the sub-folder having the name same as that of the package name (in AndroidManifest.xml file). This folder will have the activities of the application and you can analyze the code for any loophole.
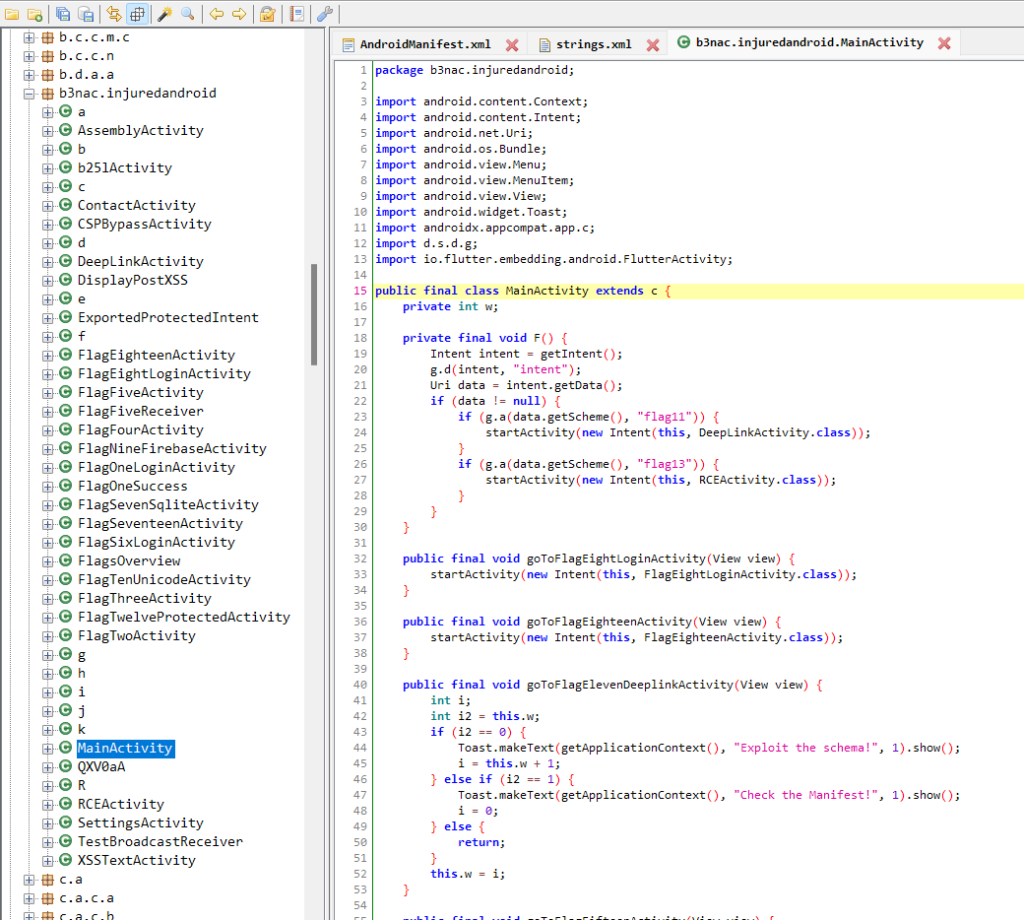
Automatic APK Static Analysis
For having the static analysis automatically, you can use MobSF and upload your APK. MobSF will analyze the APK for all the potential sensitive things and give us the summary of the findings.
Conclusion
Not necessarily, but it is a good practice to do a static analysis of the application (APK) before doing the dynamic analysis. As with static analysis, you will get a good understanding of the application’s structure, permissions, credentials (if any), and much more.
Leave a Reply